JavaScript variable hoisting explained
You may have heard of JavaScript "hoisting" variables in a function, but you're not sure what it means. You'd like to be sure, so you don't make any mistakes related to variable declarations.
"What does variable hoisting mean?"
Variable hoisting is one of JavaScript's idiosyncracies but it has a coping strategy and also upcoming pain relief in ES6.
Variable declarations moved to top of function
Variable hoisting means that all var
statements in a function are moved to the top of the function regardless of their location in the function. For example
function fn() {
var a;
console.log('hello world');
var b;
}
when run is interpreted by JavaScript as
function fn() {
var a;
var b; // hoisted
console.log('hello world');
}
Note how the declaration of variable b
has changed place.
Declaration and initialization split into two
This moving of var
statements only applies to the declaration of a variable. If there's an initialization combined with the declaration, the initialization stays where it is. This means that putting a variable declaration statement with an initializer
var string = "abc";
anywhere in function body acts as the statement is split into two and the declaration is moved to the top of the function
// to top of function
var string;
// original location
string = "abc";
Coping mechanism: declare variables at the top
A coping mechanism exists for addressing variable hoisting. Only declare variables with var
at the top of a function. This way there will not be any surprises regarding hoisting.
function fn() {
// declare var-variables here
// rest of the function logic here
}
ES6 let works more sanely
ES6 brings us two new keywords that are related to variable declaration: const
and let
. The const
keyword is used for creating values that are only assigned once. The let
keyword is used for creating block scope variables (in contrast to var
creating a function scope variable).
A variable declared using the let
keyword acts differently in the case of referencing the variable before it's initialized. Variable hoisting allows us to create code that references a variable before it is declared
function fn() {
console.log('variable a is: ' + a); // prints `undefined`
var a = "abc";
}
here the declaration of a
is hoisted to top of the function and it gets assigned the value undefined
. A variable declared using let
does get hoisted, but it will not get initialized but will produce an error if it is accessed without a value.
function fn() {
console.log('variable a is: ' + a); // "ReferenceError: a is not defined"
let a = "abc";
}
This eases us the approach of declaring variables at the top of a function, its no longer necessary for let
-declared variables.
Related articles
- Should I use === or == equality comparison operator in JavaScript?
- Understanding the prototype property in JavaScript
- Using && and || outside of if-clause
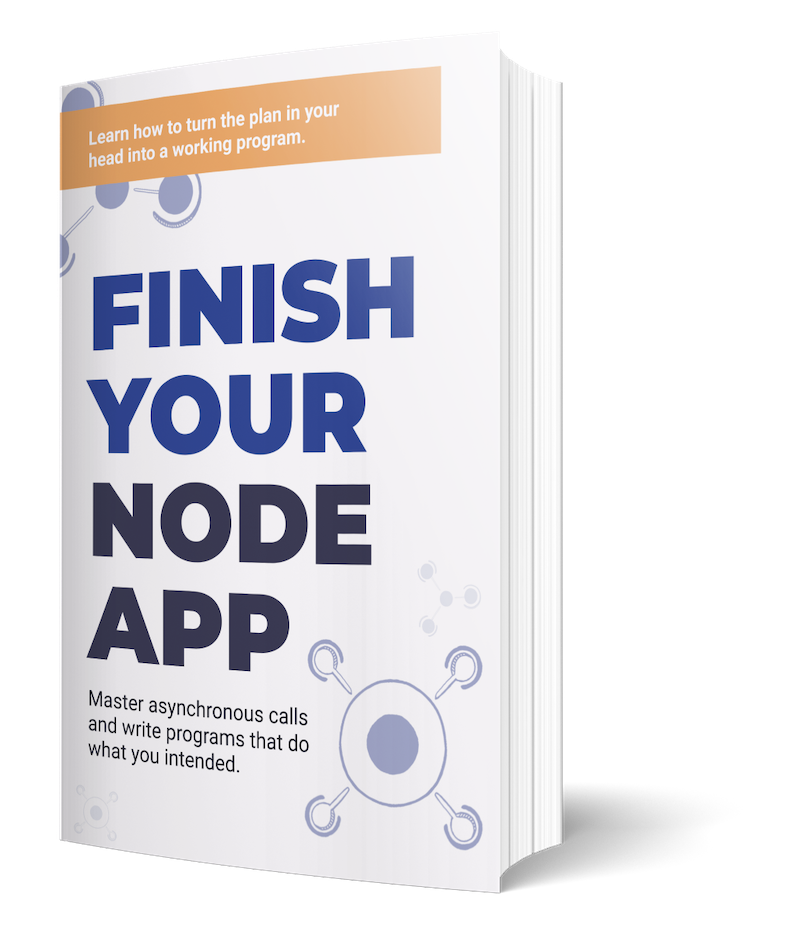
Node doesn't wait for your database call to finish?
Learn how asynchronous calls work and make your app run as you intended. Get short email course on asynchronicity and two chapters from Finish Your Node App.