Using promise.then(callback, callback) misses errors
One traditional example of using promises is
promise.then(callback, callback);
There exists one important caveat in this approach. Can you spot it?
Exception in success handler goes unnoticed
If the success handler throws exception, then it will not be caught and handled in the rejection handler as one would think.
An improved version would be to separate the error handler as its own step. Then chain that only after the success handler. This way exception in the success handler will be propagated to the correct place.
promise
.then(callback)
.catch(callback);
A real world example
This becomes important in longer promise chains. For example if renderResultPage
throws an error, it will not end up in handleError
as you might expect:
fetchUrl()
.then(parseValues)
.then(loadPreviousValuesFromDb)
.then(calculateTodaysQuote)
.then(writeBackToDb)
.then(renderResultPage, handleError)
it's better to use catch()
to avoid this
...
.then(writeBackToDb)
.then(renderResultPage)
.catch(handleError)
and catch all errors.
Test case
Mocha test case
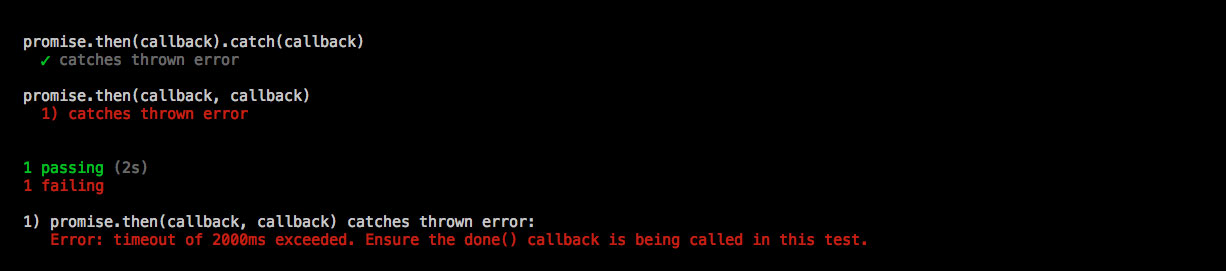
Test case available at
https://github.com/bytearcher/using-promise-then-callback-callback-misses-errors
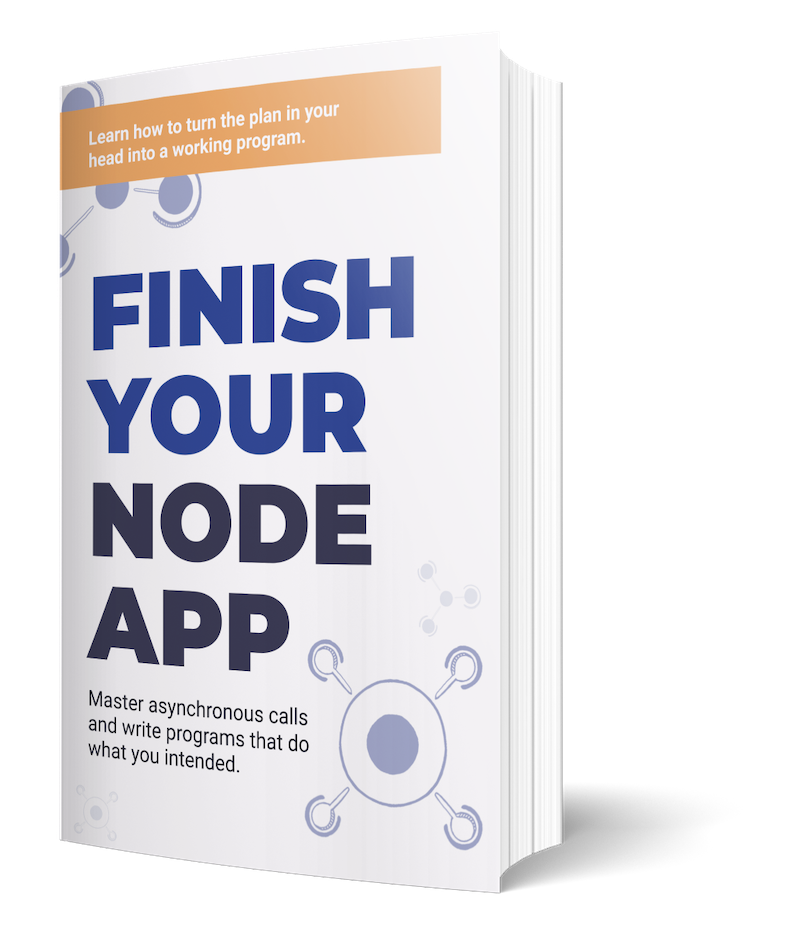
Node doesn't wait for your database call to finish?
Learn how asynchronous calls work and make your app run as you intended. Get short email course on asynchronicity and two chapters from Finish Your Node App.