Fast TDD setup for TypeScript
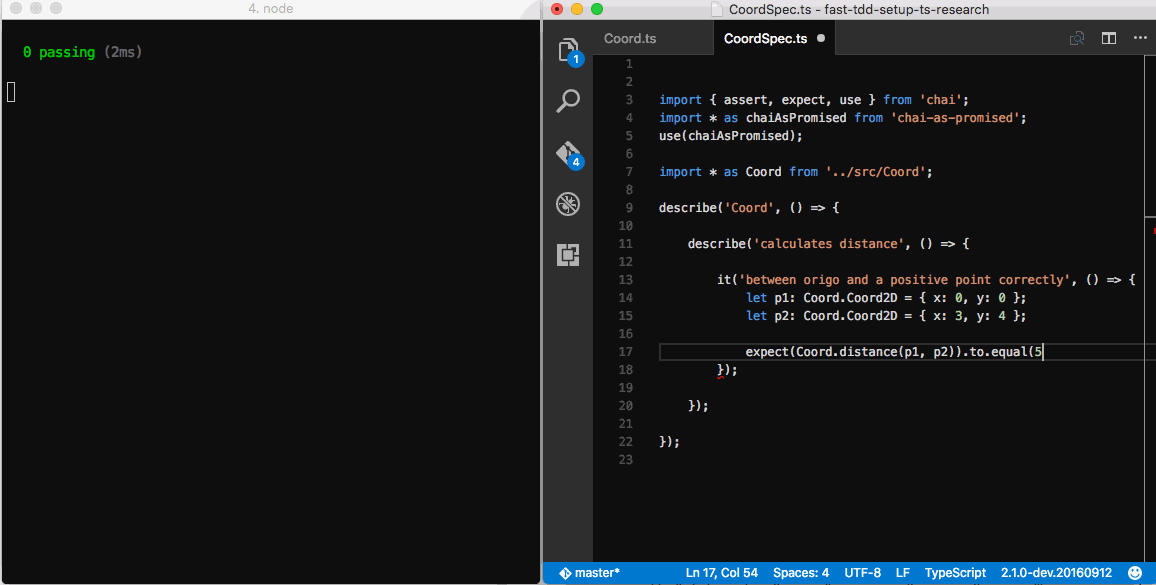
You need good tools for writing tests
Writing tests is not the most enjoyable task for many developers. Things become much easier when you have a fast response time in the edit-compile-test cycle. Combine it with a good expressive testing framework and you're off to a better start.
Test-driven development (TDD) is an approach to software development where you write unit tests along with the application code. You'd typically write an empty method stub, create a failing test that verifies not-yet-existing program logic, and then complete the program logic until your test becomes green. Lastly, you'd refactor your code before pushing your commit.
Let's create a fast TDD setup for TypeScript.
Run immediately, show only failing
Many IDEs support unit testing and test-driven development but most developers write JavaScript in plain text editors. We need a command line approach to creating a fast TDD setup.
It should
- wait for changes and run tests immediately, so we only need to save the file and test are started
- it should show only failing tests because our screens are not big enough to hold 100 successful tests
There's one annoying corner case that needs to be taken care of, too. If the previous test run has not yet completed, and a new one is started, the previous one needs to be terminated. This is something that for example grunt and mocha combination does not handle.
Fast TDD setup for TypeScript
Here's a setup that suits our requirements. It works on command line. It'll watch for changed files, then compile TypeScript and launch test runner. It'll terminate if the previous run hasn't yet exited. It'll only show failed tests.
The script uses gaze-run-interrupt
npm package to watch for changed files and fire a sequence of commands, possibly killing the previous sequence. It uses Mocha and its min-reporter to show only the failed tests.
Steps
1. Add the gaze-run-interrupt
as devDependency to your package.json
.
2. Save this in a file called tdd
and give it execution permissions. Fix paths according to your project structure.
#!/usr/bin/env node
const gaze_run_interrupt = require('gaze-run-interrupt');
gaze_run_interrupt('{src,test}/**/*.ts', [{
command: 'node_modules/.bin/tsc'
}, {
command: '../../node_modules/.bin/mocha',
args: ['--reporter', 'min'],
cwd: 'dist/js'
}]);
3. Start your TDD session by
./tdd
and start writing good quality code - with tests.
Now, try this out and drop a comment below if it improved your workflow!
Download the project files used in this article to create the fast TDD setup. Copy them and use them in your project.