How to delete a value from an array in JavaScript
This classic question pops up once in a while. Even the creator of Node.js Ryan Dahl asked this question from the audience during his Node.js presentation (an excellent one by the way).
How do I remove an element from an array?
Is the delete
operator of any use? There also exists funny named splice()
and slice()
, what about those? I want to modify the array in-place.
Use splice() to remove arbitrary item
The correct way to remove an item from an array is to use splice()
. It takes an index and amount of items to delete starting from that index.
> let array = ["a", "b", "c"];
> let index = 1;
> array.splice(index, 1);
[ 'b' ]
> array;
[ 'a', 'c' ]
Don't confuse this with its similar cousin slice()
that is used to extract a section of an array.
Use shift() to remove from beginning
If you're always interested in removing the first or the last item, then you have an alternative solution. The methods shift()
and pop()
exist precisely for this purpose. With shift()
, you can remove the first item.
> let array = ["a", "b", "c"];
> array.shift();
'a'
> array;
[ 'b', 'c' ]
Use pop() to remove from end
And with pop()
you can remove the last item.
> let array = ["a", "b", "c"];
> array.pop();
'c'
> array;
[ 'a', 'b' ]
Using delete creates empty spots
Whatever you do, don't use delete
to remove an item from an array. JavaScript language specifies that arrays are sparse, i.e., they can have holes in them.
Using delete
creates these kinds of holes. It removes an item from the array, but it doesn't update the length property. This leaves the array in a funny state that is best avoided.
> let array = ["a", "b", "c"];
> delete array[1];
> array;
[ 'a', , 'c' ]
> array.length
3
Notice the empty spot and unchanged length.
Remember this
The next time you need to remove something from an array, keep the following in mind.
Remove? | |
---|---|
An item | array.splice(index, 1) |
First item | array.shift() |
Last item | array.pop() |
What about delete? | Try to avoid delete , causes sparse arrays. |
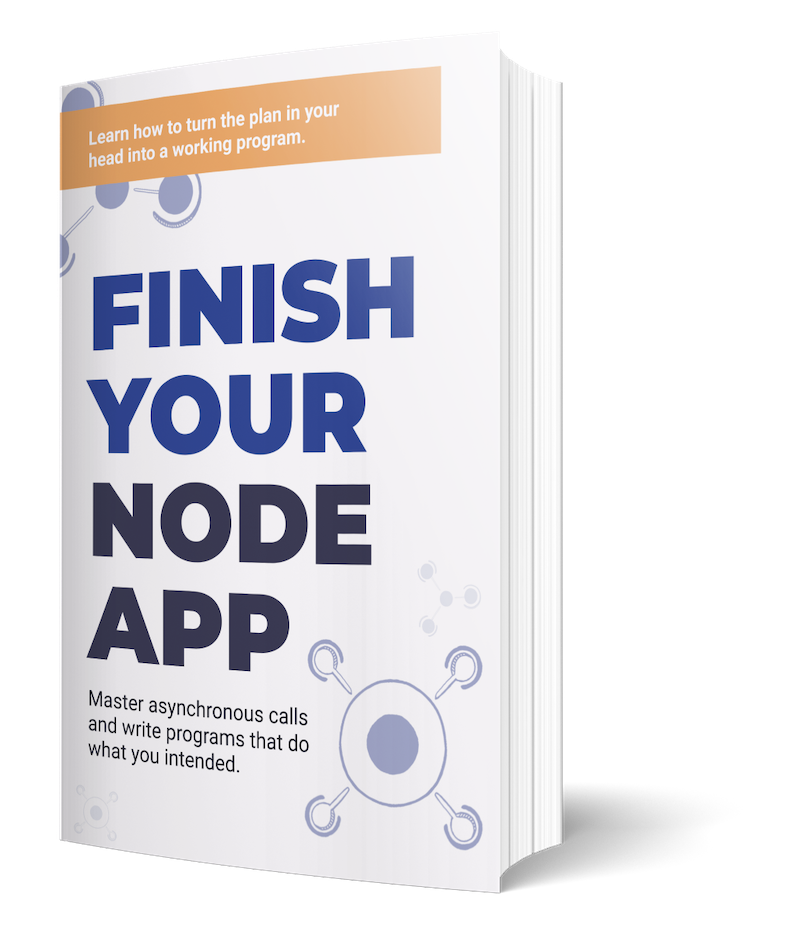
Node doesn't wait for your database call to finish?
Learn how asynchronous calls work and make your app run as you intended. Get short email course on asynchronicity and two chapters from Finish Your Node App.